Dev C++ Call Function
- Dev C++ Calling Function
- C++ Function Call By Reference
- Function In Dev C++
- C++ Function Call Operator
- Dev C++ Programs
- Dev C Call Function N By Name
- C# Call Method
A function is a block of code which only runs when it is called.
You can pass data, known as parameters, into a function.
- Calling C functions from GO. 03 Jan 2017 in Go / Interop. How to call C functions from GO. I’ve recently started learning GO and given that I’ve spent majority of my career in interop between runtimes and languages, I’m naturally curious on how you can interop between GO and other languages.
- C handles passing an array to a function in this way to save memory and time. Passing Multidimensional Array to a Function Multidimensional array can be passed in similar way as one-dimensional array.
- Deduction guides (since C17) NoteCare should be taken when a std::function whose result type is a reference is initialized from a lambda expression without a trailing-return-type. Due to the way auto deduction works, such lambda expression will always return a prvalue.
- Hey guys I'm trying to work out how to call a function in my code using a switch statement. I have tried to look for many different references but no matter what nothing seems to work if somebody c.
- Nov 13, 2014 Similarly, you should do like this for your next void functions when calling. Beside, notice about the variables, whether using value variable or reference variable. In this case, if you want to print the value of x, y, z after executing the above void function, you should declare like this: void intialize(int& x.
Functions are used to perform certain actions, and they are important for reusing code: Define the code once, and use it many times.
registercallback is wapper of registercallback to call cbproxy. Waitevent will invoke callback internally with taking argument userdata. Userdata should be passed from main. However, one another issue you meet. Rules for passing pointers between Go and C. Unfortunately again, Go can't pass pointer which is allocated in Go into C function. Function Call (C); 2 minutes to read +2; In this article. The function-call operator, invoked using parentheses, is a binary operator. Syntax primary-expression ( expression-list ) Remarks. In this context, primary-expression is the first operand, and expression-list, a possibly empty list of arguments, is the second operand.
Create a Function
C++ provides some pre-defined functions, such as main()
, which is used to execute code. But you can also create your own functions to perform certain actions.
To create (often referred to as declare) a function, specify the name of the function, followed by parentheses ():
D piano-a vst free download. 3 Sounds FX Collections Producers will also Addicted and liking the versatility of this Pack when creating Tracks from Scratch. This Tuneswill Compliment for Trancethrough to ambient, Dubstep, Progressive, Electro House, Tech House,Goa, and many others!
FMDrive 1.25 for Windows MINIMUM price to get the FMDrive package, is 15 Euros. Thank you for willing to buy the FMDrive VST Software. You will support to make the creation process going on and make those musical ideas alive! Once your payment is accepted you will receive an Email with instructions and the FMDrive software link within 3 to 7 days Max. Nov 14, 2018 64-bit 2018 2019 analog au bass best DAW delay Download easy Editor edm eq fm free free download Full fx help high sierra hip hop izotope MAC mastering microsoft mixing mojave native instruments os x osx plugin Plugins release reverb sine sound design studio synth synthesizer techno trance vst windows working. Pirate bay. Aly James Lab new release the FmDrive Sega Mega Drive – Genesis FM VST Synthesizer for Windows. This Windows 32-bit VST synth is suitable for chip music, Sega nostalgic pleasure and also a powerful FM tool for modern music production. You can design a vast range of sounds on top.
Syntax
Example Explained
myFunction()
is the name of the functionvoid
means that the function does not have a return value. You will learn more about return values later in the next chapter- inside the function (the body), add code that defines what the function should do
Call a Function
Declared functions are not executed immediately. They are 'saved for later use', and will be executed later, when they are called.
To call a function, write the function's name followed by two parentheses ()
and a semicolon ;
In the following example, myFunction()
is used to print a text (the action), when it is called:
Example
Inside main
, call myFunction()
:

void myFunction() {
cout << 'I just got executed!';
}
int main() {
myFunction(); // call the function
return 0;
}
// Outputs 'I just got executed!'
A function can be called multiple times:
Dev C++ Calling Function
Example
C++ Function Call By Reference
cout << 'I just got executed!n';
}
int main() {
myFunction();
myFunction();
myFunction();
return 0;
}
// I just got executed!
// I just got executed!
// I just got executed!
Function Declaration and Definition
A C++ function consist of two parts:
Function In Dev C++
- Declaration: the function's name, return type, and parameters (if any)
- Definition: the body of the function (code to be executed)
C++ Function Call Operator
// the body of the function (definition)
}
Note: If a user-defined function, such as myFunction()
is declared after the main()
function, an error will occur. It is because C++ works from top to bottom; which means that if the function is not declared above main()
, the program is unaware of it:
Example
myFunction();
return 0;
}
void myFunction() {
cout << 'I just got executed!';
}
// Error
Dev C++ Programs
However, it is possible to separate the declaration and the definition of the function - for code optimization.
You will often see C++ programs that have function declaration above main()
, and function definition below main()
. This will make the code better organized and easier to read:
Example
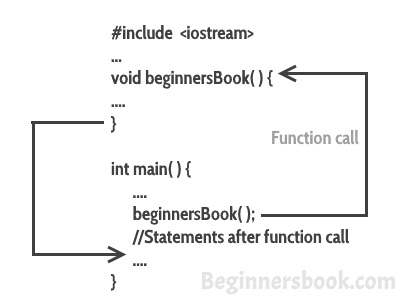
Dev C Call Function N By Name
void myFunction();
// The main method
int main() {
myFunction(); // call the function
return 0;
}
// Function definition
void myFunction() {
cout << 'I just got executed!';
}